(Dancing Lamp # 1)
Before we create a dancing lamp using our PC’s parallel port, we have to know the parallel port female pin-out (see the picture below).
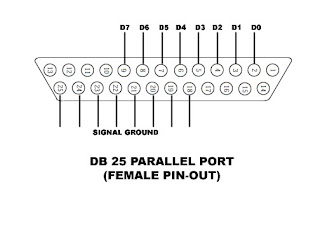
D0 is the LSB (Least Significant Bit) and D7 is the Most Significant Bit (MSB). You can send an integer type data to the D0..D7 pin-outs. And the output will be the binary form of the integer we send. For example : If we send an integer data 15 the output will be :
D7 | D6 | D5 | D4 | D3 | D2 | D1 | D0 |
LOW | LOW | LOW | LOW | HIGH | HIGH | HIGH | HIGH |
15 (byte) = 00001111 (binary)
How To Send Data To The Parallel Port ?
To send data to the parallel port I use a DLL file (Inpout32.dll) that can be downloaded at logix4u.net, and it’s free for non-commercial manner. Place this file in C:\WINDOWS\system or in the same folder with you project you’re going to create.
To use this file you can see my previous tutorial about using a DLL file. This DLL file contains a function called Out32(). The syntax of this function :
Out32(portaddress,data);
Usually, the port address is $378 (you can check the BIOS setting of your PC)
Example program :
unit Unit1;
interface
uses
Windows, Messages, SysUtils, Classes, Graphics, Controls, Forms, Dialogs,
StdCtrls;
type
TForm1 = class(TForm)
Edit1: TEdit;
Label1: TLabel;
Button1: TButton;
procedure FormActivate(Sender: TObject);
procedure Button1Click(Sender: TObject);
private
{ Private declarations }public
{ Public declarations }end;
var
Form1: TForm1;
implementation
{$R *.DFM}function Out32(wAddr:word;bOut:byte):byte; stdcall; external 'inpout32.dll';
procedure TForm1.FormActivate(Sender: TObject);
begin
Edit1.Text := '';
Edit1.SetFocus;
end;
procedure TForm1.Button1Click(Sender: TObject);
begin
Out32($378,StrToInt(Edit1.Text));
end;
end.
You can download the complete code here.
Go to next tutorial (LED Circuit)